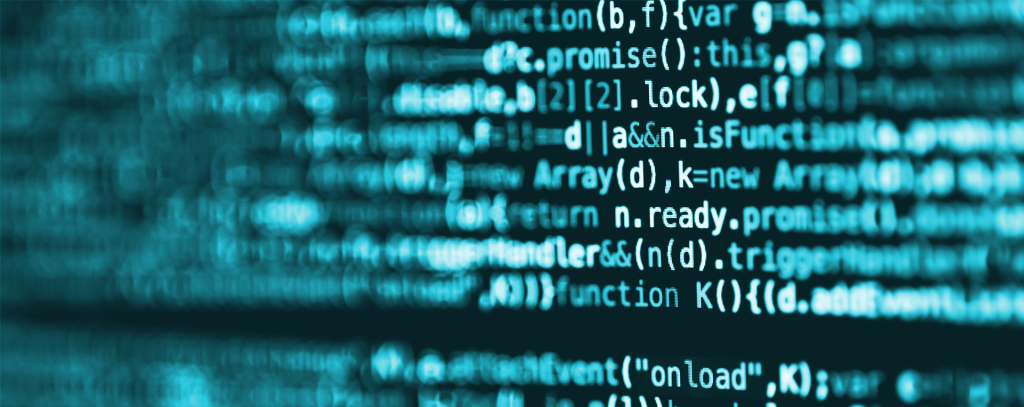
In the world of programming, code is king. It is the driving force behind everything we do, the foundation on which we build our applications. But not all code is created equal. Some code is well-structured, easy to read, and efficient, while other code is bloated, hard to maintain, and difficult to understand. One of the key principles of writing high-quality code is the DRY (Don’t Repeat Yourself) principle. In this article, we’ll take a closer look at what DRY code is, why it matters, and how to apply it in your own development projects.
What is DRY Code?
The DRY principle is a software development principle that was first coined by software engineer Andy Hunt and Dave Thomas in their book, “The Pragmatic Programmer.” At its core, the DRY principle states that “Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.”
In other words, DRY code is code that avoids repetition. If you find yourself copying and pasting the same block of code over and over again, you’re violating the DRY principle. Instead, you should strive to write code that is modular, reusable, and easy to maintain.
Why Does DRY Code Matter?
There are several reasons why writing DRY code is important. First and foremost, DRY code is easier to read and understand. When you’re working on a project that spans thousands of lines of code, it can be overwhelming to try to understand what’s going on if the same block of code is repeated multiple times throughout the project. By avoiding repetition and adhering to the DRY principle, you can make your code more readable and easier to understand.
In addition to improving readability, writing DRY code can also save you time and effort in the long run. When you write code that is modular and reusable, you can easily repurpose that code in other parts of your project or in future projects. This can save you a lot of time and effort compared to writing new code from scratch every time you need to perform a similar task.
Finally, DRY code is often more efficient than code that violates the DRY principle. When you avoid repetition and write modular code, you can often eliminate unnecessary calculations or processing that can slow down your application. This can result in faster load times, better performance, and a better user experience.
How to Write DRY Code:
Now that we understand what DRY code is and why it’s important, let’s take a look at some best practices for writing DRY code.
- Use Functions and Classes:
One of the best ways to avoid repetition in your code is to use functions and classes. By defining a function or class that performs a specific task, you can reuse that code throughout your project without having to copy and paste it every time you need it.
- Avoid Hard-Coded Values:
Another common source of repetition in code is hard-coded values. For example, if you’re working on a web application and you’ve hard-coded the URL of your API endpoint in multiple places throughout your code, you’re violating the DRY principle. Instead, you should define that URL as a variable or constant and reference it throughout your code.
- Use Templates:
If you find yourself writing the same HTML or CSS code over and over again, you can use templates to avoid repetition. For example, if you’re building a web application with multiple pages, you can define a template for the header and footer of each page and then include that template on each page. This can save you a lot of time and effort compared to copying and pasting the same code on each page.
- Refactor Your Code:
Finally, if you’ve already written code that violates the DRY principle, you can refactor your code to make it more modular and reusable. Refactoring is the process of making changes to your code without changing its external behavior. This can involve extracting common code into functions or classes, replacing hard-coded values with variables or constants, or using templates to avoid repetition.
Conclusion:
In summary, writing DRY code is an important principle of software development. By avoiding repetition and writing modular, reusable code, you can improve the readability, efficiency, and maintainability of your applications. Some best practices for writing DRY code include using functions and classes, avoiding hard-coded values, using templates, and refactoring existing code. By following these principles, you can write code that is easier to read, easier to maintain, and more efficient. So next time you’re working on a programming project, remember to keep DRY in mind!